The behavior is certainly annoying but nothing that could not be solved without a userscript. Below is a script that preserves text entered in any input of the flag modal (including custom reasons in submodals) while allowing the modal to be closed upon clicking away from it.
Script Preview:
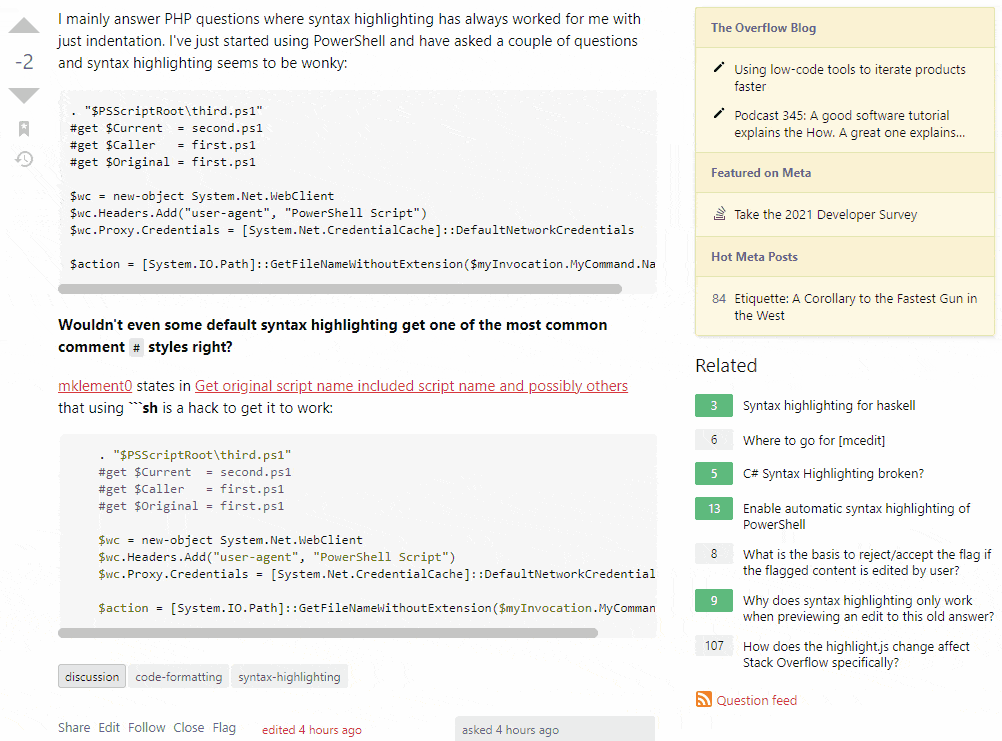
TypeScript source:
type Inputs = HTMLTextAreaElement | HTMLInputElement;
type Data = { [name: string]: string };
((_w, d) => {
const flagModalQueries = ["#popup-flag-post", "#popup-close-question"];
const submitBtnQuery = ".js-popup-submit";
const skey = "_flag-overcharged";
const save = (data: Data) => {
try {
localStorage.setItem(skey, JSON.stringify(data));
} catch (error) {
console.debug(`failed to persist input data: ${error}`);
}
};
const load = () => JSON.parse(localStorage.getItem(skey) || "{}");
const findRecord = (records: MutationRecord[], skipped: number[]) => {
return records.find(({ addedNodes }) =>
[...addedNodes].some(
(node) =>
!skipped.includes(node.nodeType) &&
flagModalQueries.some((query) =>
(<HTMLElement>node).matches(query)
)
)
);
};
const throttle = <T extends (...args: any[]) => any>(
cbk: T,
period = 100
) => {
let throttled = false;
return (...args: Parameters<T>) => {
if (!throttled) {
throttled = true;
setTimeout(() => (throttled = false), period);
return cbk(...args);
}
};
};
const savedData: Data = load();
const skippedNodeTypes = [Node.COMMENT_NODE, Node.TEXT_NODE];
const obs = new MutationObserver((records) => {
const record = findRecord(records, skippedNodeTypes);
if (!record) return;
const {
addedNodes: [flagModule],
} = record;
const modal = <HTMLDivElement>flagModule;
modal.addEventListener(
"input",
throttle(({ target }) => {
const { name, value } = <HTMLInputElement>target;
savedData[name] = value;
save(savedData);
})
);
modal.addEventListener("click", ({ target }) => {
if (!(<HTMLElement>target).matches(submitBtnQuery)) return;
const inputs = [
...modal.querySelectorAll<Inputs>("input, textarea"),
];
inputs.forEach(
(input) => (input.value = savedData[input.name] = "")
);
});
Object.entries(savedData).forEach(([name, value]) => {
const input = modal.querySelector<Inputs>(`[name=${name}]`);
if (!input) return;
input.value = value;
});
});
obs.observe(d, {
subtree: true,
childList: true,
});
})(window, document);
Compiled and minified version:
"use strict";(e=>{const s=["#popup-flag-post","#popup-close-question"],r="_flag-overcharged";const n=JSON.parse(localStorage.getItem(r)||"{}"),t=[Node.COMMENT_NODE,Node.TEXT_NODE],o=new MutationObserver(e=>{var o,e=(e=e,o=t,e.find(({addedNodes:e})=>[...e].some(t=>!o.includes(t.nodeType)&&s.some(e=>t.matches(e)))));if(e){var{addedNodes:[e]}=e;const a=e;a.addEventListener("input",((t,o=100)=>{let a=!1;return(...e)=>{if(!a)return a=!0,setTimeout(()=>a=!1,o),t(...e)}})(({target:e})=>{var{name:t,value:e}=e;n[t]=e,(e=>{try{localStorage.setItem(r,JSON.stringify(e))}catch(e){console.debug(`failed to persist input data: ${e}`)}})(n)})),a.addEventListener("click",({target:e})=>{if(e.matches(".js-popup-submit")){const t=[...a.querySelectorAll("input, textarea")];t.forEach(e=>e.value=n[e.name]="")}}),Object.entries(n).forEach(([e,t])=>{const o=a.querySelector(`[name=${e}]`);o&&(o.value=t)})}});o.observe(e,{subtree:!0,childList:!0})})((window,document));
Compiled and minified ES5 version:
"use strict";var __read=this&&this.__read||function(e,r){var t="function"==typeof Symbol&&e[Symbol.iterator];if(!t)return e;var n,a,o=t.call(e),i=[];try{for(;(void 0===r||0<r--)&&!(n=o.next()).done;)i.push(n.value)}catch(e){a={error:e}}finally{try{n&&!n.done&&(t=o.return)&&t.call(o)}finally{if(a)throw a.error}}return i},__spreadArray=this&&this.__spreadArray||function(e,r){for(var t=0,n=r.length,a=e.length;t<n;t++,a++)e[a]=r[t];return e};!function(e){var a=["#popup-flag-post","#popup-close-question"],o="_flag-overcharged",i=JSON.parse(localStorage.getItem(o)||"{}"),r=[Node.COMMENT_NODE,Node.TEXT_NODE];new MutationObserver(function(e){var t,n,e=(t=r,e.find(function(e){e=e.addedNodes;return __spreadArray([],__read(e)).some(function(r){return!t.includes(r.nodeType)&&a.some(function(e){return r.matches(e)})})}));e&&((n=__read(e.addedNodes,1)[0]).addEventListener("input",function(t,n){void 0===n&&(n=100);var a=!1;return function(){for(var e=[],r=0;r<arguments.length;r++)e[r]=arguments[r];if(!a)return a=!0,setTimeout(function(){return a=!1},n),t.apply(void 0,__spreadArray([],__read(e)))}}(function(e){var r=e.target,e=r.name,r=r.value;i[e]=r,function(e){try{localStorage.setItem(o,JSON.stringify(e))}catch(e){console.debug("failed to persist input data: "+e)}}(i)})),n.addEventListener("click",function(e){e.target.matches(".js-popup-submit")&&__spreadArray([],__read(n.querySelectorAll("input, textarea"))).forEach(function(e){return e.value=i[e.name]=""})}),Object.entries(i).forEach(function(e){var r=__read(e,2),e=r[0],r=r[1],e=n.querySelector("[name="+e+"]");e&&(e.value=r)}))}).observe(e,{subtree:!0,childList:!0})}((window,document));
Unminified version with userscript headers: snippet is for the spoiler only
// ==UserScript==
// @author Oleg Valter
// @description Enhancements for flagging experience
// @homepage https://github.com/userscripters/flag-overcharged#readme
// @match ://*.askubuntu.com/*
// @match ://*.mathoverflow.net/*
// @match ://*.serverfault.com/*
// @match ://*.stackapps.com/*
// @match ://*.stackexchange.com/*
// @match ://*.stackoverflow.com/*
// @match ://*.superuser.com/*
// @name flag-overcharged
// @source git+https://github.com/userscripters/flag-overcharged.git
// @supportURL https://github.com/userscripters/flag-overcharged/issues
// @version 1.1.0
// ==/UserScript==
"use strict";
((_w, d) => {
const flagModalQueries = ["#popup-flag-post", "#popup-close-question"];
const submitBtnQuery = ".js-popup-submit";
const skey = "_flag-overcharged";
const save = (data) => {
try {
localStorage.setItem(skey, JSON.stringify(data));
}
catch (error) {
console.debug(`failed to persist input data: ${error}`);
}
};
const load = () => JSON.parse(localStorage.getItem(skey) || "{}");
const findRecord = (records, skipped) => {
return records.find(({ addedNodes }) => [...addedNodes].some((node) => !skipped.includes(node.nodeType) &&
flagModalQueries.some((query) => node.matches(query))));
};
const throttle = (cbk, period = 100) => {
let throttled = false;
return (...args) => {
if (!throttled) {
throttled = true;
setTimeout(() => (throttled = false), period);
return cbk(...args);
}
};
};
const savedData = load();
const skippedNodeTypes = [Node.COMMENT_NODE, Node.TEXT_NODE];
const obs = new MutationObserver((records) => {
const record = findRecord(records, skippedNodeTypes);
if (!record)
return;
const { addedNodes: [flagModule], } = record;
const modal = flagModule;
modal.addEventListener("input", throttle(({ target }) => {
const { name, value } = target;
savedData[name] = value;
save(savedData);
}));
modal.addEventListener("click", ({ target }) => {
if (!target.matches(submitBtnQuery))
return;
const inputs = [
...modal.querySelectorAll("input, textarea"),
];
inputs.forEach((input) => (input.value = savedData[input.name] = ""));
});
Object.entries(savedData).forEach(([name, value]) => {
const input = modal.querySelector(`[name=${name}]`);
if (!input)
return;
input.value = value;
});
});
obs.observe(d, {
subtree: true,
childList: true,
});
})(window, document);
localStorage
/sessionStorage
with persistent text. There is also a friendlier solution of not removing the modal from the DOM but collapsing it instead thus keeping the text should the user open the modal again. P.s. yivi, have another +1 for the request